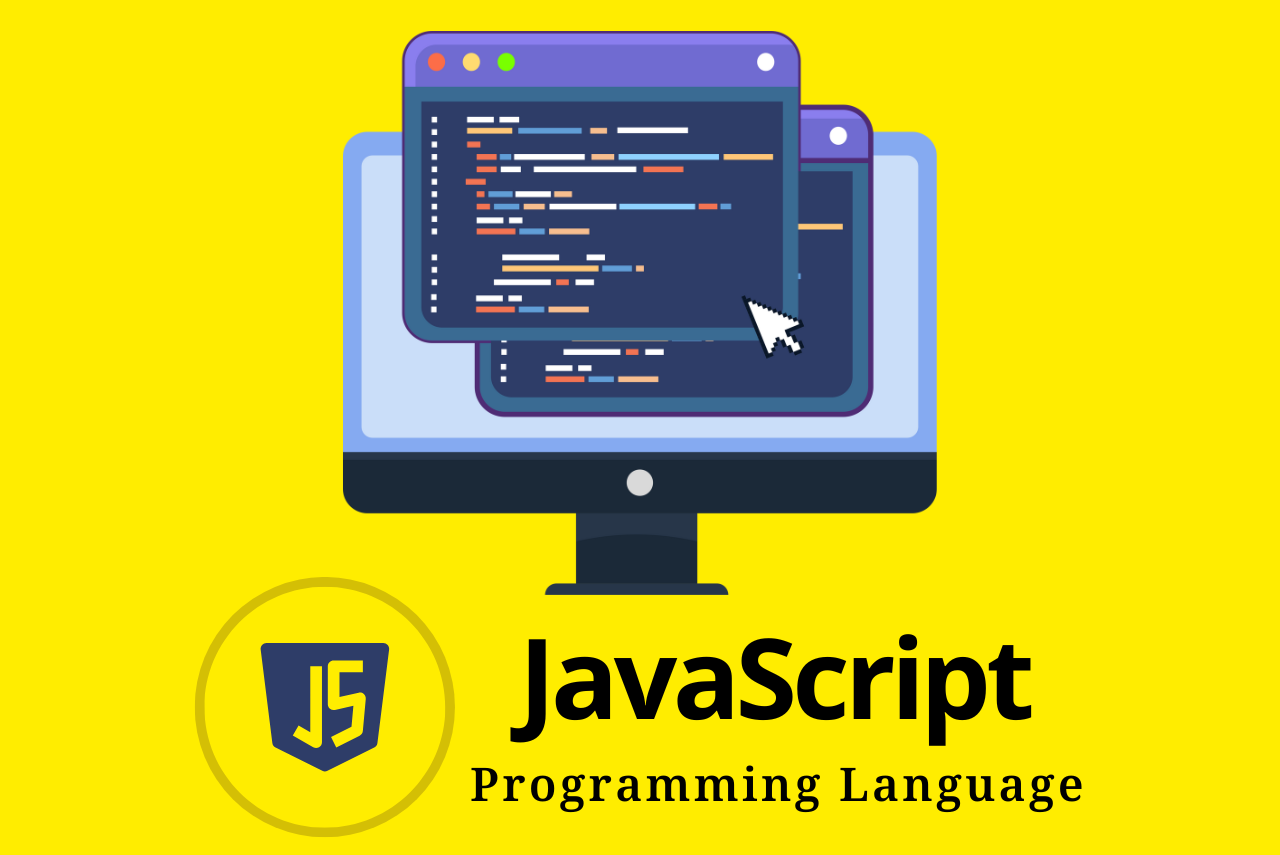
Java Script
assurance
Course Overview
JavaScript is one of the most powerful and versatile programming languages in the world. Whether you’re building interactive websites, developing server-side applications, or creating games, JavaScript is the foundation that makes it all possible.
Welcome to the JavaScript course! This course is designed to provide you with a solid foundation in JavaScript, the programming language of the web. Whether you’re a beginner or have some coding experience, this course will guide you through JavaScript essentials and prepare you for building interactive web applications.
What you are going to learn?
1. Introduction to Java
- What is Java?
- Setting up Java Development Environment
- Basic Java Syntax
2. Data Types and Variables
- Primitive Data Types
- Variables
- Type Casting
3. Control Flow Statements
- Conditionals
- Loops
- Break and Continue
4. Object-Oriented Programming (OOP)
- Classes and Objects
- Constructors
- Methods
- Encapsulation
- Inheritance
- Polymorphism
- Abstraction
- Interfaces
5. Data Structures
- Arrays
- Strings
- Collections Framework
6. Exception Handling
- Exceptions
- Custom Exceptions
- Throwing Exceptions
7. File I/O (Input/Output)
- Reading and Writing Files
- Serialization
8. Multithreading and Concurrency
- Threads
- Concurrency
- Executor Service
9. Java APIs and Libraries
- Standard Library
- Java Streams API
- Date and Time API
10. Database Connectivity (JDBC)
- JDBC (Java Database Connectivity)
- Prepared Statements
- Transaction Management
11. JavaFX (for GUI Applications)
- Creating GUIs
- Layouts
12. Unit Testing
- JUnit
- Mocking
13. Advanced Java Features
- Lambda Expressions
- Generics
- Reflection
- Annotations
14. Design Patterns
- Common Design Patterns
15. Deployment and Packaging
- JAR Files
- Java Web Applications
16. Frameworks and Libraries
- Spring Framework
- Hibernate
17. Java Best Practices
- Code Quality
- Refactoring
18. Final Projects
- Build Real-World Applications
What You Will Be Able to Do After Learning JavaScript?
1. Build Interactive Web Applications
- Develop dynamic and responsive web applications that update in real-time based on user interactions.
2. Create Single Page Applications (SPAs)
- Build SPAs using frameworks like React, Angular, or Vue.js, ensuring seamless user experiencesul>
3. Work with Backend Development
- Use Node.js to create server-side applications and manage databases with Express and MongoDB.
4. Manage State and Data Flow
- Utilize tools like Redux, Context API, or React Query to manage application state efficiently.
5. Enhance Performance and Optimization
- Optimize web applications using lazy loading, code splitting, and performance best practices.
6. Implement Authentication and Security
- Integrate authentication mechanisms like JWT, OAuth, and Firebase Authentication for secure applications.
7. Write Maintainable Code
- Follow best coding practices, modularization, and clean code principles to improve maintainability.
8. Debug and Test JavaScript Code
- Use debugging tools and frameworks like Jest, Mocha, and Cypress to ensure application reliability.
9. Work with APIs and Fetch Data
- Connect to RESTful APIs and GraphQL endpoints to fetch and display dynamic data.
10. Deploy and Manage Applications
- Deploy applications to hosting services like Netlify, Vercel, or AWS with CI/CD integration.
Course Duration
6 months
Mode of Course
- Online
- In-Person
Course Content
FAQS
No prior programming experience is required. However, basic computer literacy and problem-solving skills will be helpful.
The course duration is typically 6 months.
Yes, the course includes multiple hands-on projects.
Yes, you will receive a certificate of completion after successfully finishing the course.
This course prepares you for roles such as:
You can pause the course and resume within a specified period. Contact support for further details.
No, the course starts with the basics, ensuring beginners can comfortably learn and progress.